Svelte Device Mockups - Flowbite
Use the device mockups component to add content and screenshot previews of your application inside phone and tablet frames coded with Tailwind CSS and Flowbite
The device mockup component can be used to feature a preview and screenshot of your application as if you would already use it on a mobile phone and it’s a great use case for hero and CTA sections.
This component is built using only the utility classes from Tailwind CSS and has built-in dark mode support so it’s easy to customize, it loads very fast and integrates perfectly with Tailwind CSS and Flowbite.
You can choose from multiple examples of mockups including phone, tablet, laptop, and even desktop devices with iOS or Android support.
Setup #
- Svelte
<script>
import { DeviceMockup } from 'flowbite-svelte';
</script>
Default mockup #
Use this example to show a standard phone mockup based on Tailwind CSS and add your app screenshot inside of it with dark mode support included.
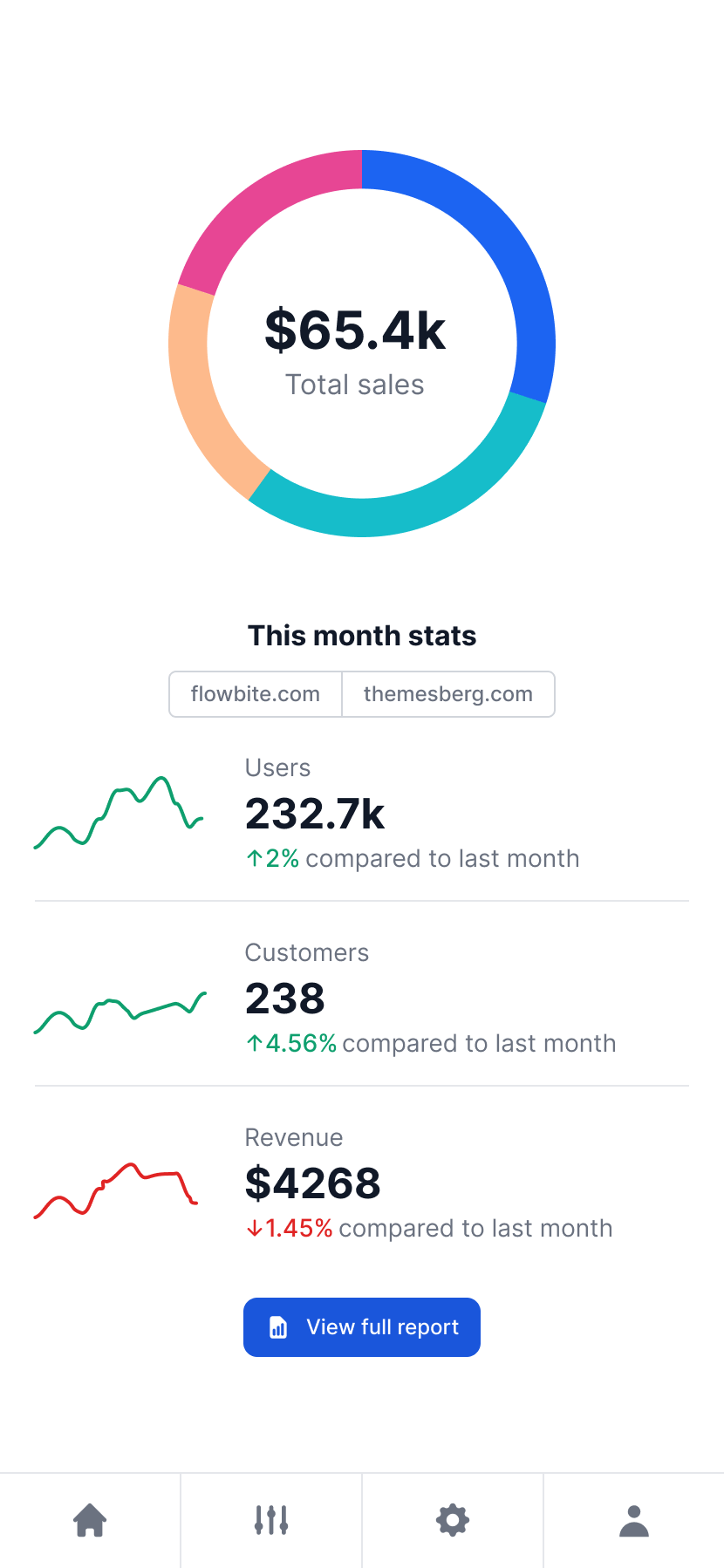
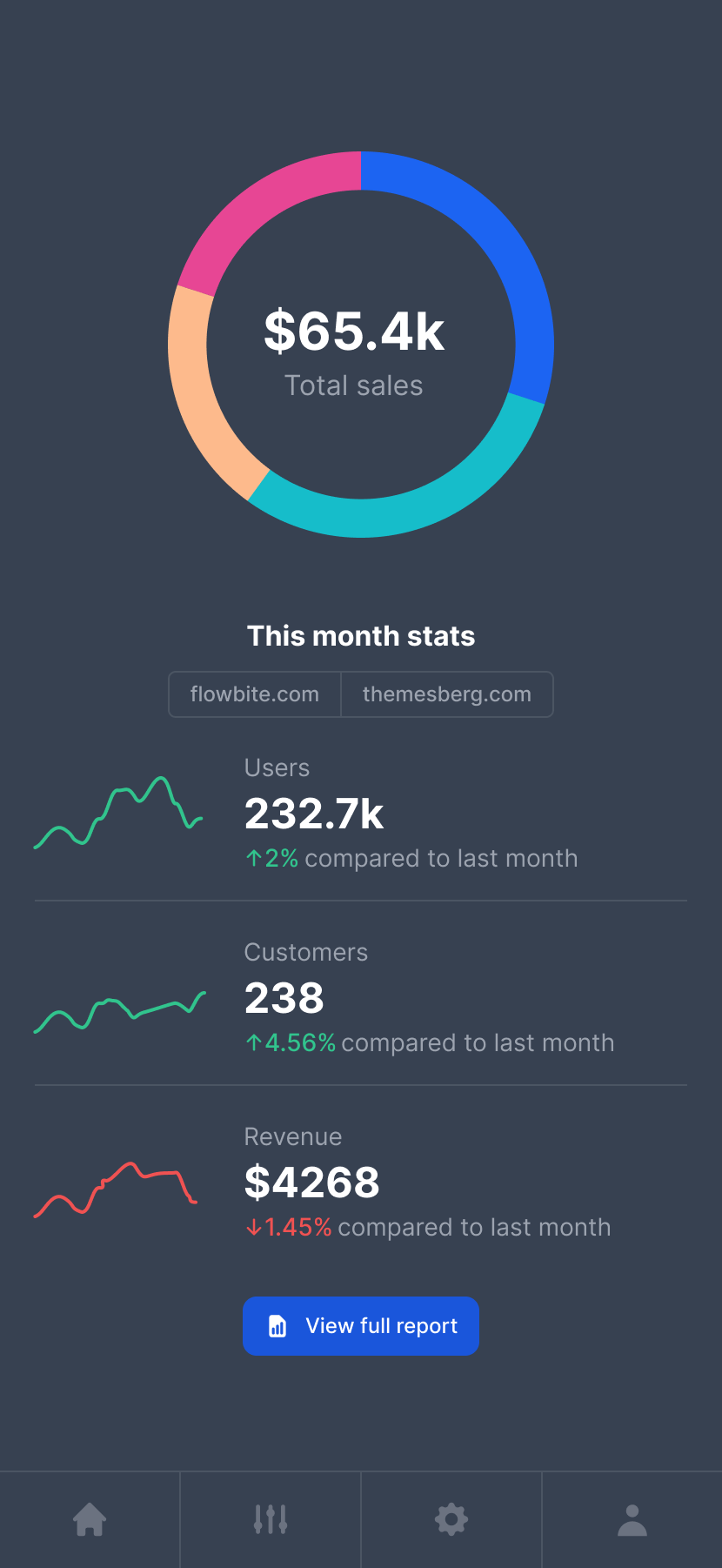
- Svelte
<script>
import { DeviceMockup } from 'flowbite-svelte';
</script>
<DeviceMockup>
<img
src="https://flowbite.s3.amazonaws.com/blocks/marketing-ui/hero/mockup-1-light.png"
class="dark:hidden w-[272px] h-[572px]"
alt="default example 1" />
<img
src="https://flowbite.s3.amazonaws.com/blocks/marketing-ui/hero/mockup-1-dark.png"
class="hidden dark:block w-[272px] h-[572px]"
alt="default example 2" />
</DeviceMockup>
iPhone 12 mockup (iOS) #
Use this example to clearly show that the preview of your application is being used on an iPhone with iOS.
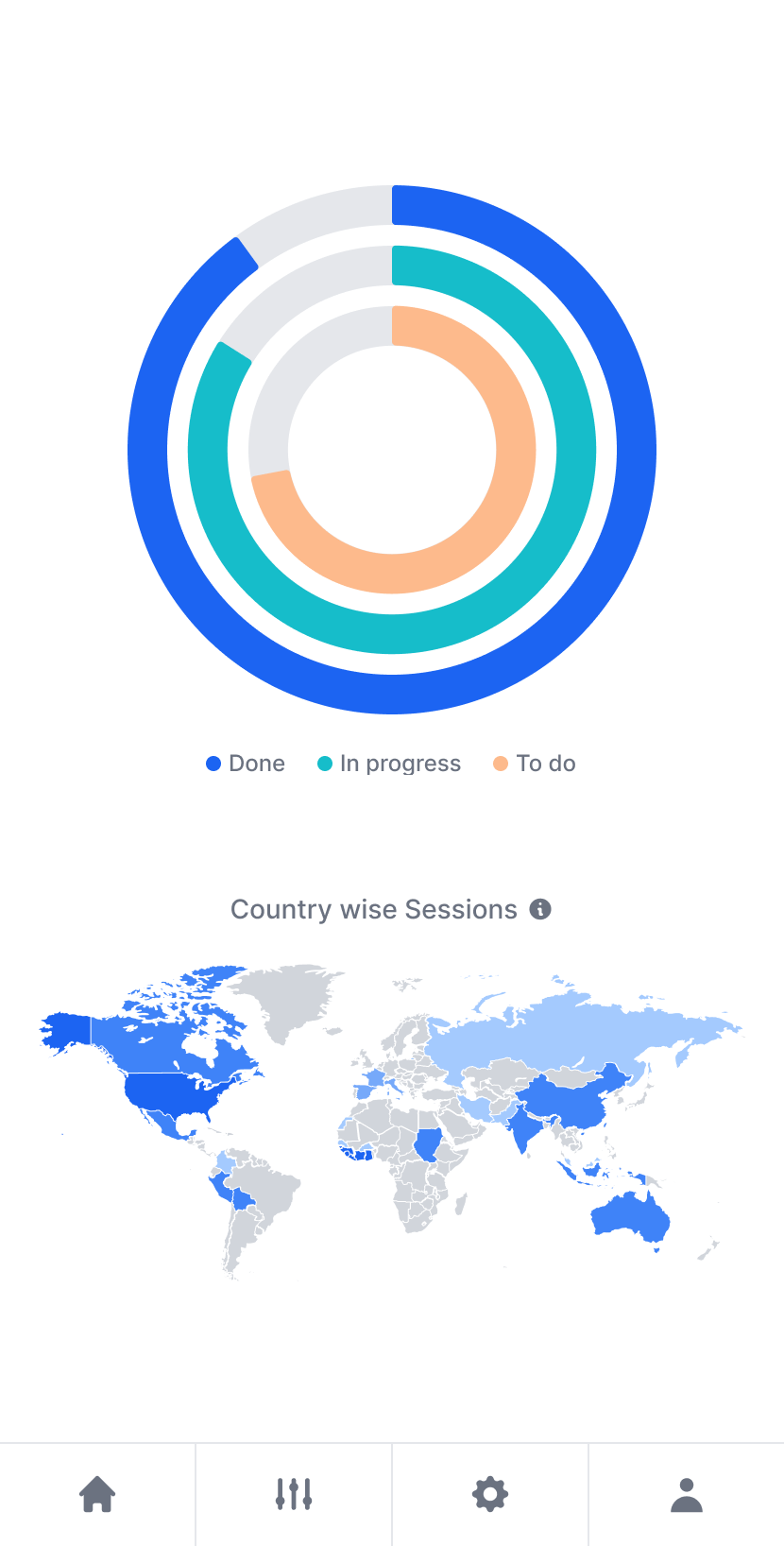
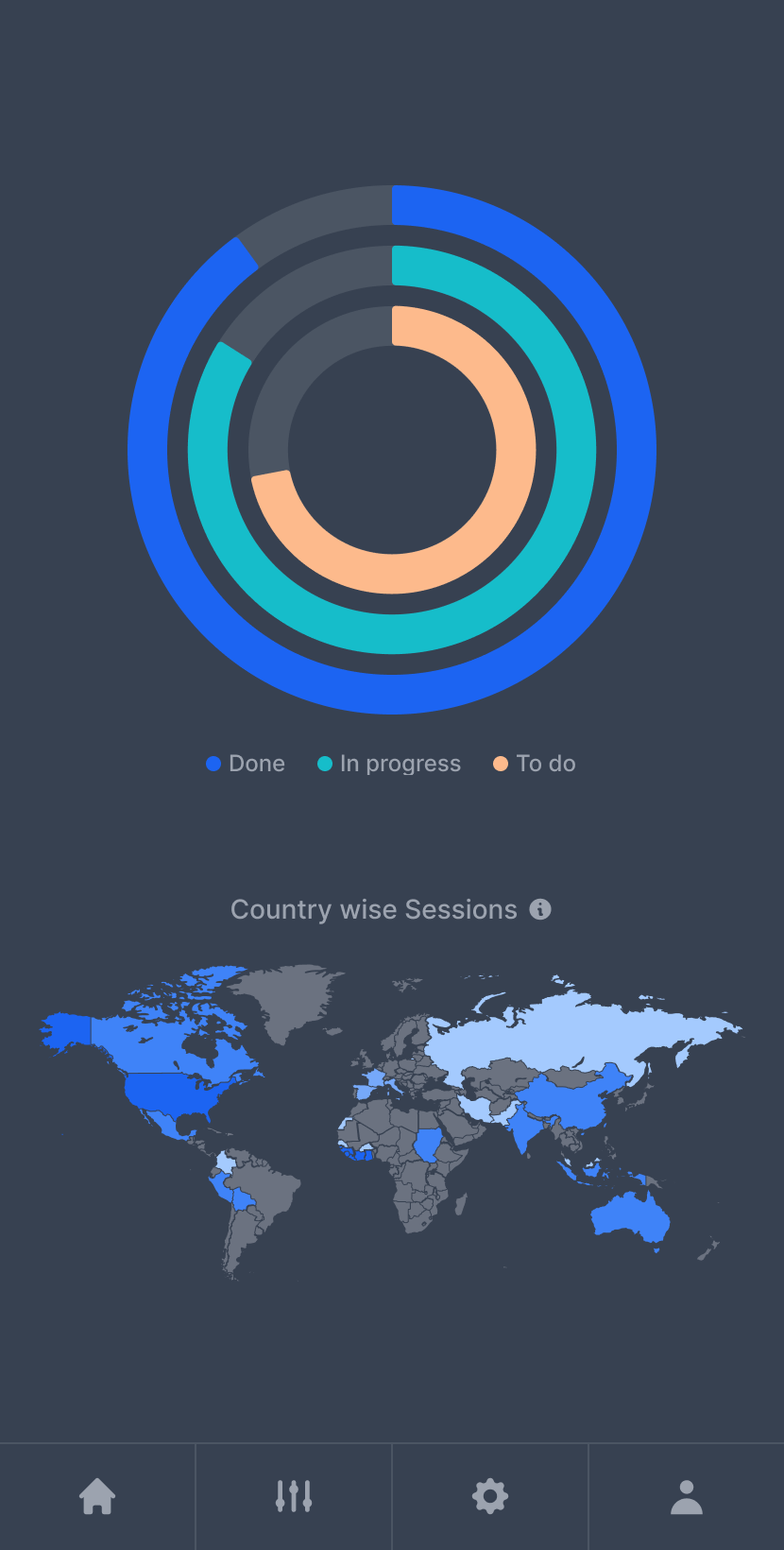
- Svelte
<script>
import { DeviceMockup } from 'flowbite-svelte';
</script>
<DeviceMockup device="ios">
<img
src="https://flowbite.s3.amazonaws.com/blocks/marketing-ui/hero/mockup-2-light.png"
class="dark:hidden w-[272px] h-[572px]"
alt="ios example 1" />
<img
src="https://flowbite.s3.amazonaws.com/blocks/marketing-ui/hero/mockup-2-dark.png"
class="hidden dark:block w-[272px] h-[572px]"
alt="ios example 2" />
</DeviceMockup>
Google Pixel mockup (Android) #
Use this alternative phone mockup example if you want to feature previews for android gadgets.
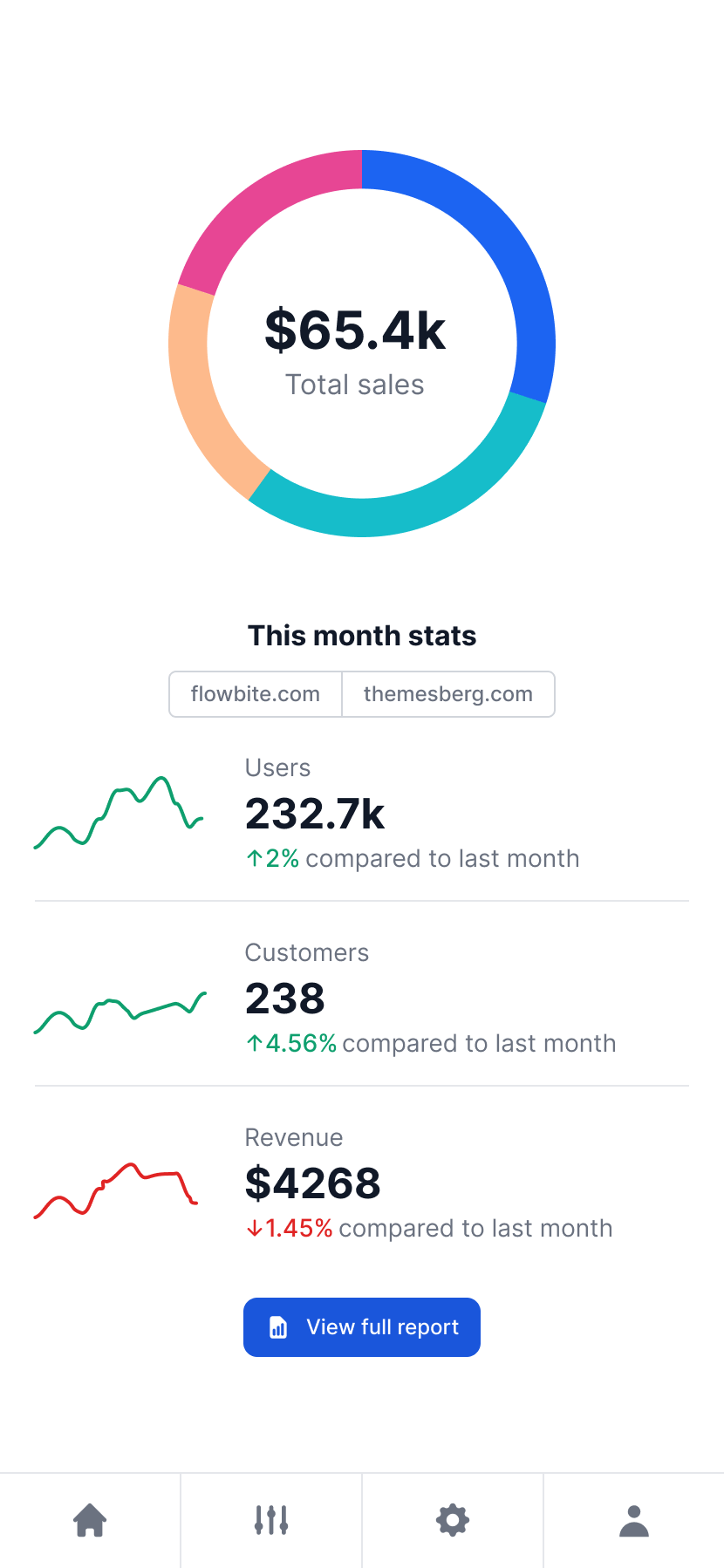
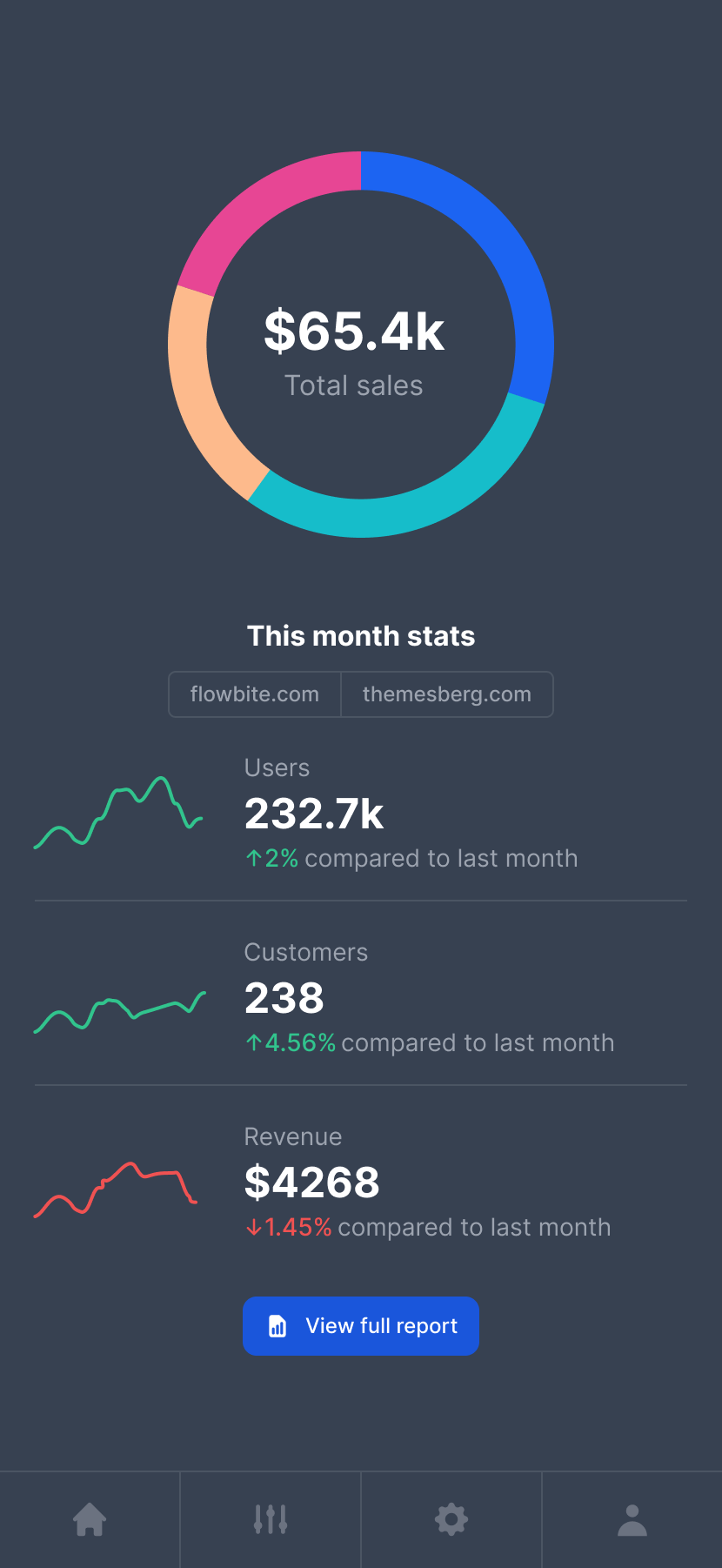
- Svelte
<script>
import { DeviceMockup } from 'flowbite-svelte';
</script>
<DeviceMockup device="android">
<img
src="https://flowbite.s3.amazonaws.com/blocks/marketing-ui/hero/mockup-1-light.png"
class="dark:hidden w-[272px] h-[572px]"
alt="android example 1" />
<img
src="https://flowbite.s3.amazonaws.com/blocks/marketing-ui/hero/mockup-1-dark.png"
class="hidden dark:block w-[272px] h-[572px]"
alt="android example 2" />
</DeviceMockup>
Tablet mockup #
This component can be used to show an application preview inside of a responsive tablet mockup.
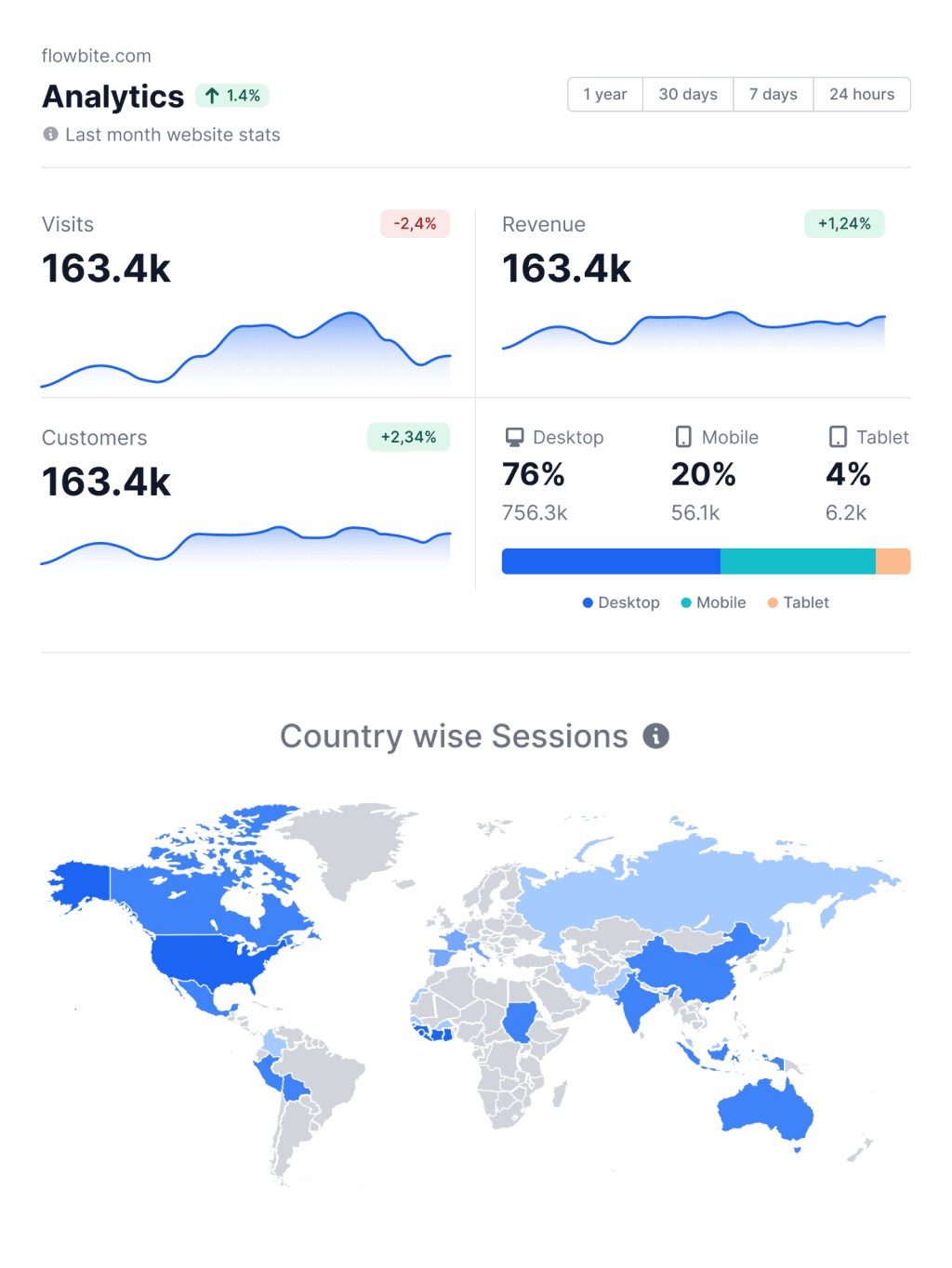
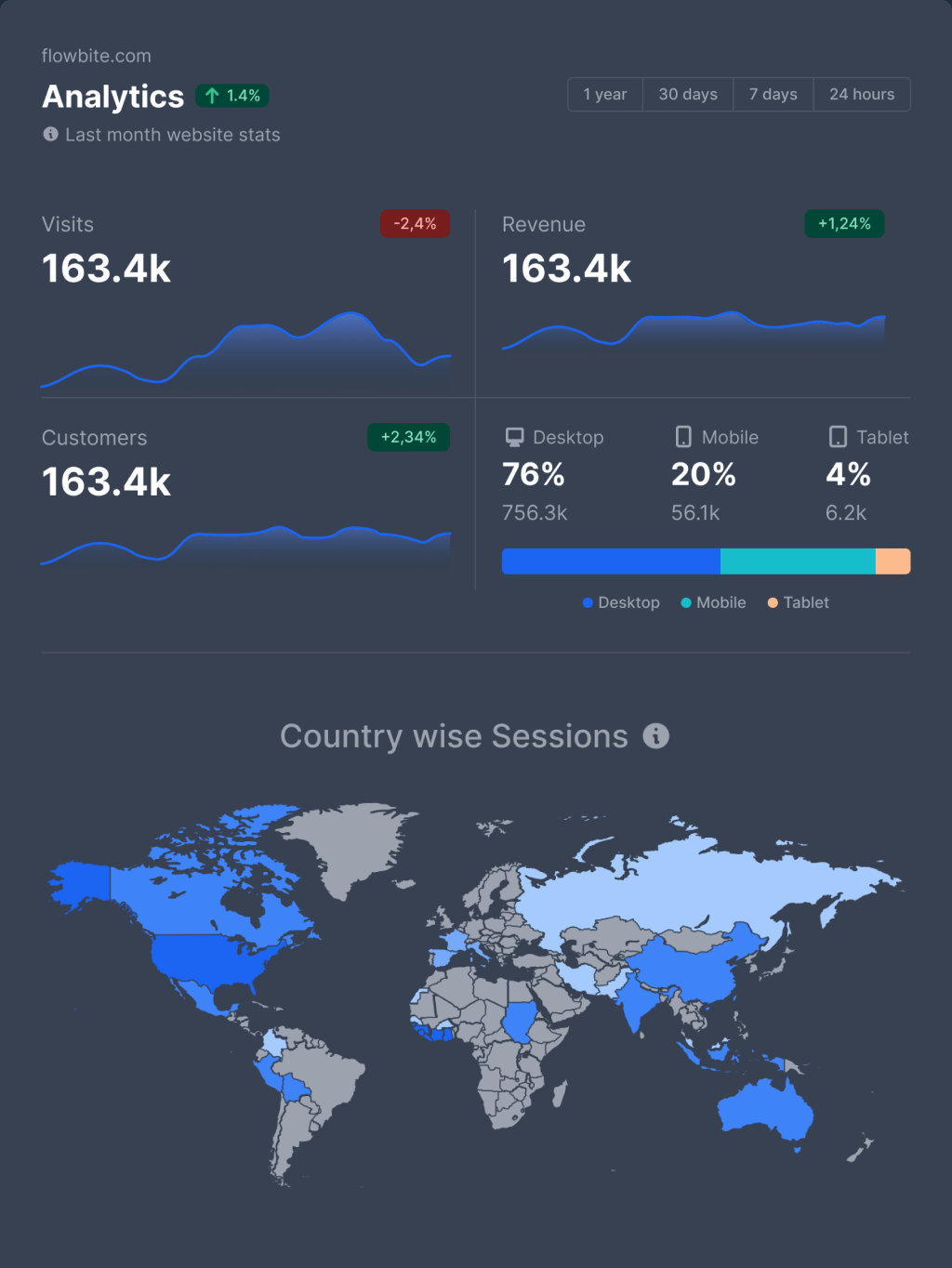
- Svelte
<script>
import { DeviceMockup } from 'flowbite-svelte';
</script>
<DeviceMockup device="tablet">
<img
src="https://flowbite.s3.amazonaws.com/docs/device-mockups/tablet-mockup-image.png"
class="dark:hidden h-[426px] md:h-[654px]"
alt="tablet example 1" />
<img
src="https://flowbite.s3.amazonaws.com/docs/device-mockups/tablet-mockup-image-dark.png"
class="hidden dark:block h-[426px] md:h-[654px]"
alt="tablet example 2" />
</DeviceMockup>
Laptop mockup #
This example can be used to show a screenshot of your application inside a laptop mockup.
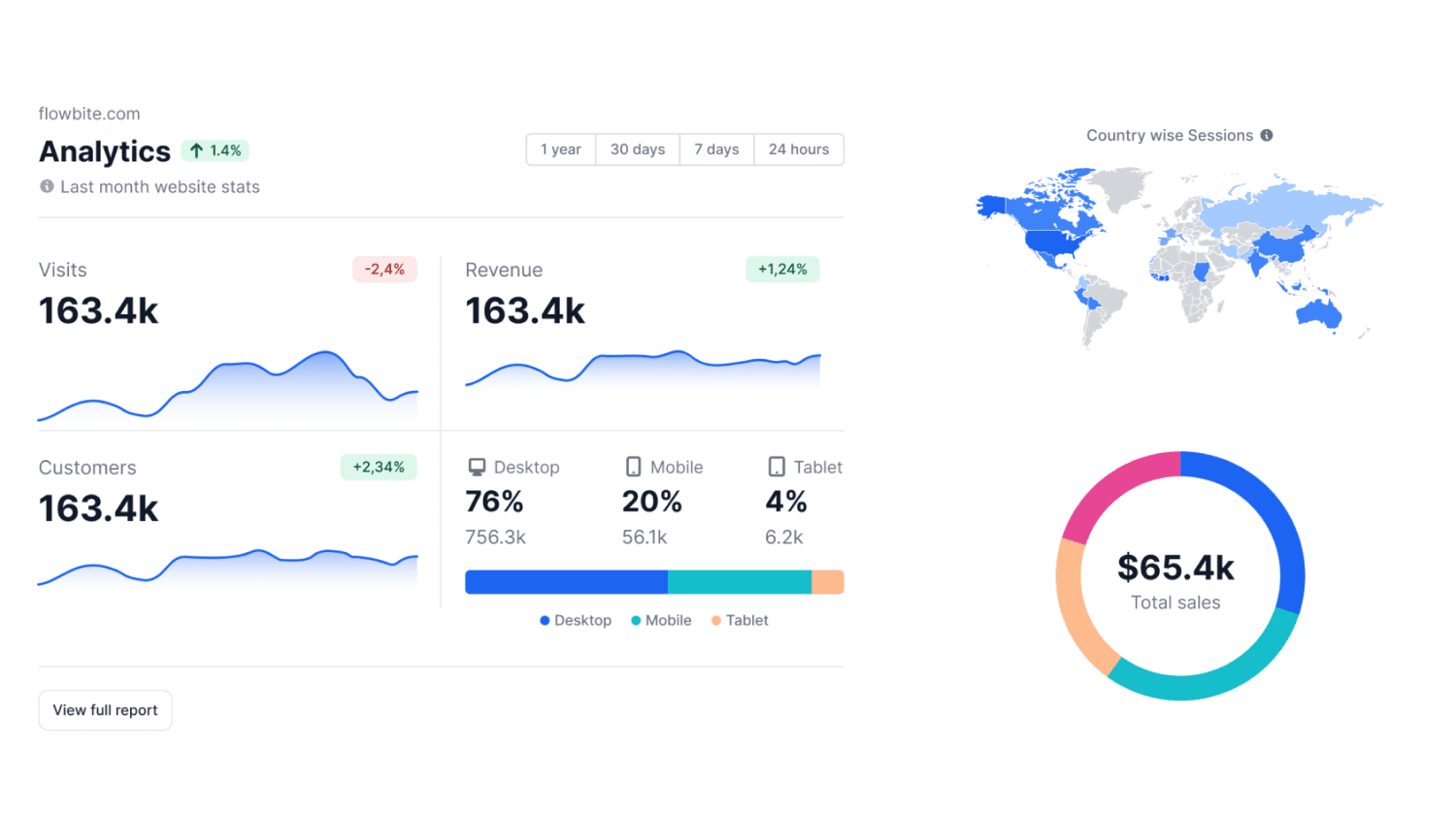
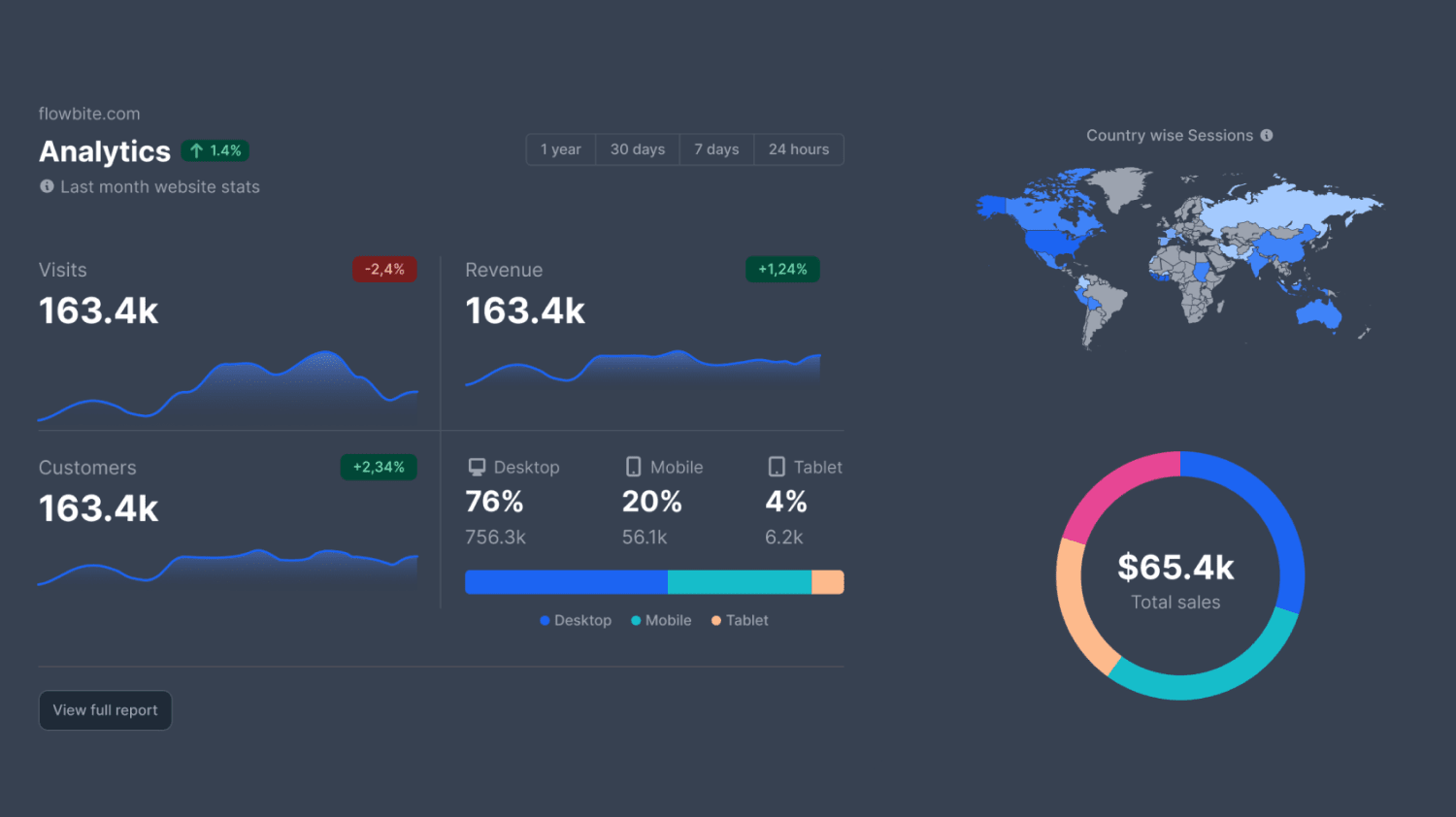
- Svelte
<script>
import { DeviceMockup } from 'flowbite-svelte';
</script>
<DeviceMockup device="laptop">
<img
src="https://flowbite.s3.amazonaws.com/docs/device-mockups/laptop-screen.png"
class="dark:hidden h-[156px] md:h-[278px] w-full rounded-xl"
alt="laptop example 1" />
<img
src="https://flowbite.s3.amazonaws.com/docs/device-mockups/laptop-screen-dark.png"
class="hidden dark:block h-[156px] md:h-[278px] w-full rounded-lg"
alt="laptop example 2" />
</DeviceMockup>
Desktop mockup #
Use this example to show a preview of your applicaiton inside a desktop device such as an iMac.
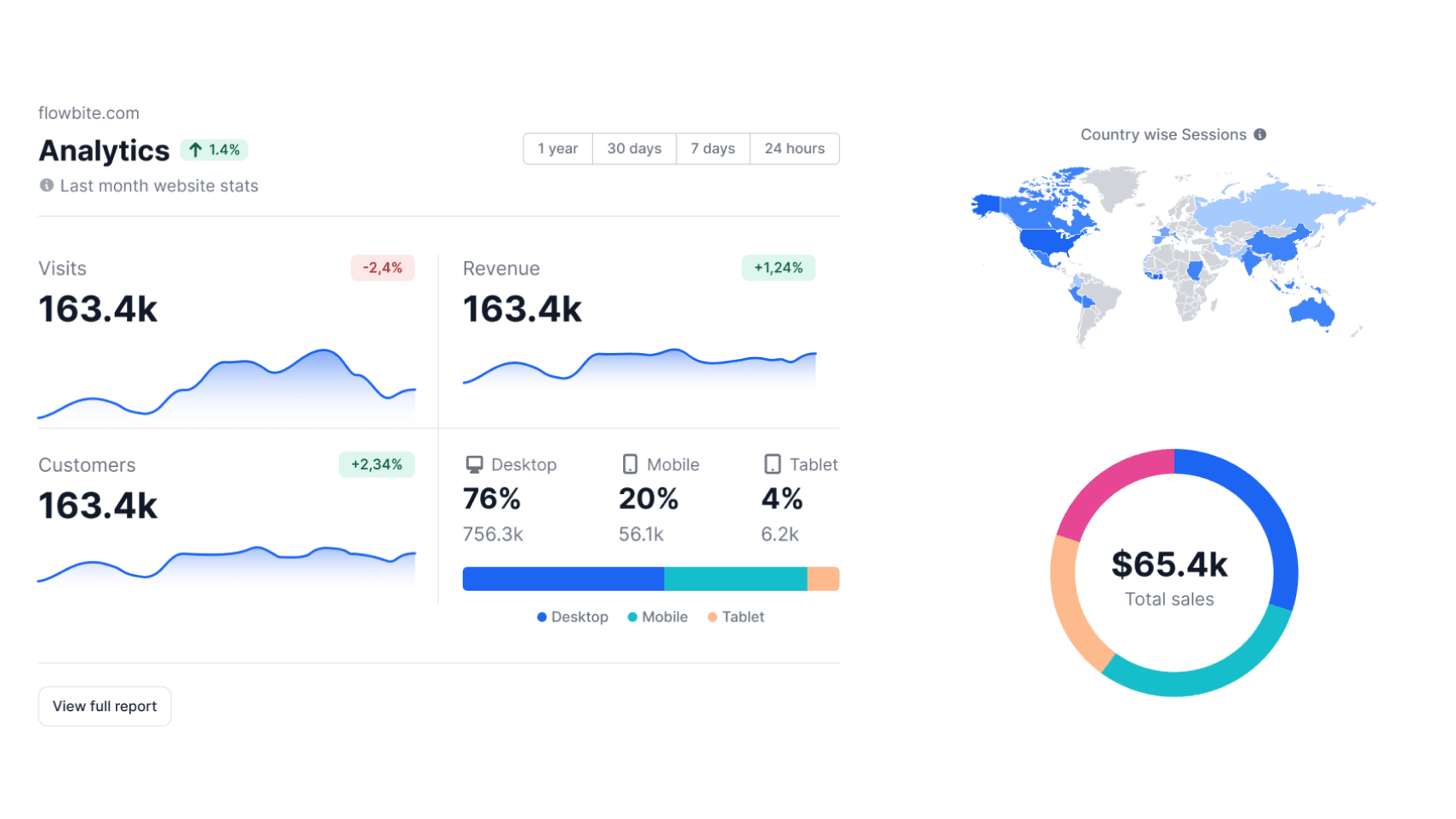
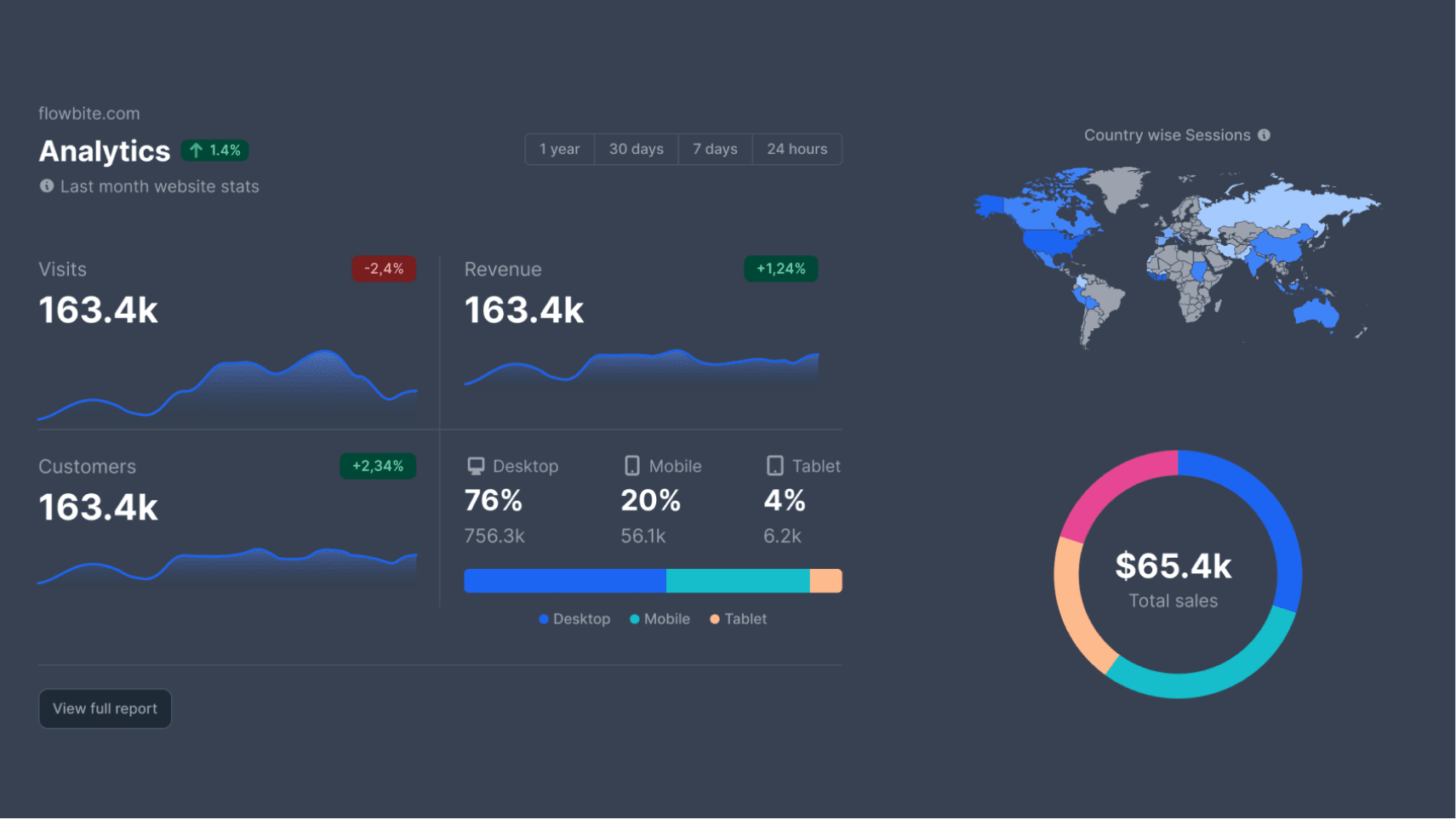
- Svelte
<script>
import { DeviceMockup } from 'flowbite-svelte';
</script>
<DeviceMockup device="desktop">
<img
src="https://flowbite.s3.amazonaws.com/docs/device-mockups/screen-image-imac.png"
class="dark:hidden h-[140px] md:h-[262px] w-full rounded-xl"
alt="desktop example 1" />
<img
src="https://flowbite.s3.amazonaws.com/docs/device-mockups/screen-image-imac-dark.png"
class="hidden dark:block h-[140px] md:h-[262px] w-full rounded-xl"
alt="desktop example 2" />
</DeviceMockup>
Smartwatch mockup #
This component can be used to showcase applications built for smartwatches.
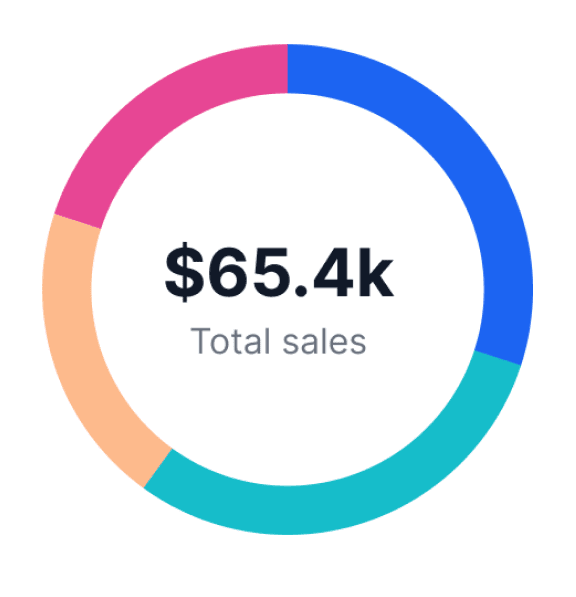
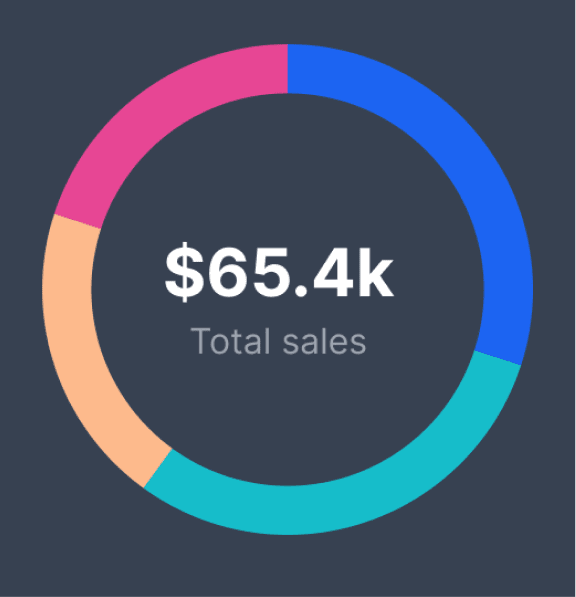
- Svelte
<script>
import { DeviceMockup } from 'flowbite-svelte';
</script>
<DeviceMockup device="smartwatch">
<img
src="https://flowbite.s3.amazonaws.com/docs/device-mockups/watch-screen-image.png"
class="dark:hidden h-[193px] w-[188px"
alt="smartwatch example 1" />
<img
src="https://flowbite.s3.amazonaws.com/docs/device-mockups/watch-screen-image-dark.png"
class="hidden dark:block h-[193px] w-[188px]"
alt="smartwatch example 2" />
</DeviceMockup>
Props #
The component has the following props, type, and default values. See types page for type information.
DeviceMockup #
- Use the
class
prop to overwrite the outer div class.
Name | Type | Default |
---|---|---|
device | 'default' | 'ios' | 'android' | 'tablet' | 'laptop' | 'desktop' | 'smartwatch' | 'default' |
androidDiv | string | 'relative mx-auto border-gray-800 dark:border-gray-800 bg-gray-800 border-[14px] rounded-xl h-[600px] w-[300px] shadow-xl' |
androidSlot | string | 'rounded-xl overflow-hidden w-[272px] h-[572px] bg-white dark:bg-gray-800' |
androidTop | string | 'w-[148px] h-[18px] bg-gray-800 top-0 rounded-b-[1rem] left-1/2 -translate-x-1/2 absolute' |
androidLeftTop | string | 'h-[32px] w-[3px] bg-gray-800 absolute -left-[17px] top-[72px] rounded-l-lg' |
androidLeftMid | string | 'h-[46px] w-[3px] bg-gray-800 absolute -left-[17px] top-[124px] rounded-l-lg' |
androidLeftBot | string | 'h-[46px] w-[3px] bg-gray-800 absolute -left-[17px] top-[178px] rounded-l-lg' |
androidRight | string | 'h-[64px] w-[3px] bg-gray-800 absolute -right-[17px] top-[142px] rounded-r-lg' |
defaultDiv | string | 'relative mx-auto border-gray-800 dark:border-gray-800 bg-gray-800 border-[14px] rounded-[2.5rem] h-[600px] w-[300px]' |
defaultSlot | string | 'rounded-[2rem] overflow-hidden w-[272px] h-[572px] bg-white dark:bg-gray-800' |
defaultTop | string | 'h-[32px] w-[3px] bg-gray-800 dark:bg-gray-800 absolute -left-[17px] top-[72px] rounded-l-lg' |
defaultLeftTop | string | 'h-[46px] w-[3px] bg-gray-800 dark:bg-gray-800 absolute -left-[17px] top-[124px] rounded-l-lg' |
defaultLeftBot | string | 'h-[46px] w-[3px] bg-gray-800 dark:bg-gray-800 absolute -left-[17px] top-[178px] rounded-l-lg' |
defaultRight | string | 'h-[64px] w-[3px] bg-gray-800 dark:bg-gray-800 absolute -right-[17px] top-[142px] rounded-r-lg' |
desktopInner | string | 'rounded-xl overflow-hidden h-[140px] md:h-[262px]' |
desktopBot | string | 'relative mx-auto bg-gray-900 dark:bg-gray-700 rounded-b-xl h-[24px] max-w-[301px] md:h-[42px] md:max-w-[512px]' |
desktopBotUnder | string | 'relative mx-auto bg-gray-800 rounded-b-xl h-[55px] max-w-[83px] md:h-[95px] md:max-w-[142px]' |
destopDiv | string | 'relative mx-auto border-gray-800 dark:border-gray-800 bg-gray-800 border-[16px] rounded-t-xl h-[172px] max-w-[301px] md:h-[294px] md:max-w-[512px]' |
desktopSlot | string | 'rounded-xl overflow-hidden h-[140px] md:h-[262px]' |
iosDiv | string | 'relative mx-auto border-gray-800 dark:border-gray-800 bg-gray-800 border-[14px] rounded-[2.5rem] h-[600px] w-[300px] shadow-xl' |
iosSlot | string | 'rounded-[2rem] overflow-hidden w-[272px] h-[572px] bg-white dark:bg-gray-800' |
iosTop | string | 'w-[148px] h-[18px] bg-gray-800 top-0 rounded-b-[1rem] left-1/2 -translate-x-1/2 absolute' |
iosLeftTop | string | 'h-[46px] w-[3px] bg-gray-800 absolute -left-[17px] top-[124px] rounded-l-lg' |
iosLeftBot | string | 'h-[46px] w-[3px] bg-gray-800 absolute -left-[17px] top-[178px] rounded-l-lg' |
iosRight | string | 'h-[64px] w-[3px] bg-gray-800 absolute -right-[17px] top-[142px] rounded-r-lg' |
laptopDiv | string | 'relative mx-auto border-gray-800 dark:border-gray-800 bg-gray-800 border-[8px] rounded-t-xl h-[172px] max-w-[301px] md:h-[294px] md:max-w-[512px]' |
laptopSlot | string | 'rounded-lg overflow-hidden h-[156px] md:h-[278px] bg-white dark:bg-gray-800' |
laptopInner | string | 'rounded-lg overflow-hidden h-[156px] md:h-[278px] bg-white dark:bg-gray-800' |
laptopBot | string | 'relative mx-auto bg-gray-900 dark:bg-gray-700 rounded-b-xl rounded-t-sm h-[17px] max-w-[351px] md:h-[21px] md:max-w-[597px]' |
laptopBotCen | string | 'absolute left-1/2 top-0 -translate-x-1/2 rounded-b-xl w-[56px] h-[5px] md:w-[96px] md:h-[8px] bg-gray-800' |
smartwatchDiv | string | 'relative mx-auto bg-gray-800 dark:bg-gray-700 rounded-t-[2.5rem] h-[63px] max-w-[133px]' |
smartwatchSlot | string | 'rounded-[2rem] overflow-hidden h-[193px] w-[188px]' |
smartRightTop | string | 'h-[41px] w-[6px] bg-gray-800 dark:bg-gray-800 absolute -right-[16px] top-[40px] rounded-r-lg' |
smartRightBot | string | 'h-[32px] w-[6px] bg-gray-800 dark:bg-gray-800 absolute -right-[16px] top-[88px] rounded-r-lg' |
smartTop | string | 'relative mx-auto border-gray-900 dark:bg-gray-800 dark:border-gray-800 border-[10px] rounded-[2.5rem] h-[213px] w-[208px]' |
smartBot | string | 'relative mx-auto bg-gray-800 dark:bg-gray-700 rounded-b-[2.5rem] h-[63px] max-w-[133px]' |
smartwatchInner | string | 'rounded-[2rem] overflow-hidden h-[193px] w-[188px]' |
tabletDiv | string | 'relative mx-auto border-gray-800 dark:border-gray-800 bg-gray-800 border-[14px] rounded-[2.5rem] h-[454px] max-w-[341px] md:h-[682px] md:max-w-[512px]' |
tabletSlot | string | 'rounded-[2rem] overflow-hidden h-[426px] md:h-[654px] bg-white dark:bg-gray-800' |
tabletLeftTop | string | 'h-[32px] w-[3px] bg-gray-800 dark:bg-gray-800 absolute -left-[17px] top-[72px] rounded-l-lg' |
tabletLeftMid | string | 'h-[46px] w-[3px] bg-gray-800 dark:bg-gray-800 absolute -left-[17px] top-[124px] rounded-l-lg' |
tabletLeftBot | string | 'h-[46px] w-[3px] bg-gray-800 dark:bg-gray-800 absolute -left-[17px] top-[178px] rounded-l-lg' |
tabletRight | string | 'h-[64px] w-[3px] bg-gray-800 dark:bg-gray-800 absolute -right-[17px] top-[142px] rounded-r-lg' |
DefaultMockup #
- Use the
classDefaultDiv
prop to overwritedefaultDiv
. - Use the
classDefaultSlot
prop to overwritedefaultSlot
. - Use the
classDefaultTop
prop to overwritedefaultTop
. - Use the
classDefaultLeftTop
prop to overwritedefaultLeftTop
. - Use the
classDefaultLeftBot
prop to overwritedefaultLeftBot
. - Use the
classDefaultRight
prop to overwritedefaultRight
.
Name | Type | Default |
---|---|---|
defaultTop | string | |
defaultLeftTop | string | |
defaultLeftBot | string | |
defaultRight | string |
Android #
- Use the
classAndroidDiv
prop to overwriteandroidDiv
. - Use the
classAndroidSlot
prop to overwriteandroidSlot
. - Use the
classAndroidTop
prop to overwriteandroidTop
. - Use the
classAndroidLeftTop
prop to overwriteandroidLeftTop
. - Use the
classAndroidLeftMid
prop to overwriteandroidLeftMid
. - Use the
classAndroidLeftBot
prop to overwriteandroidLeftBot
. - Use the
classAndroidRight
prop to overwriteandroidRight
.
Name | Type | Default |
---|---|---|
androidTop | string | |
androidLeftTop | string | |
androidLeftMid | string | |
androidLeftBot | string | |
androidRight | string |
Desktop #
- Use the
classDesktopInner
prop to overwritedesktopInner
. - Use the
classDesktopBot
prop to overwritedesktopBot
. - Use the
classDesktopBotUnder
prop to overwritedesktopBotUnder
. - Use the
classDestopDiv
prop to overwritedestopDiv
. - Use the
classDesktopSlot
prop to overwritedesktopSlot
.
Ios #
- Use the
classIosDiv
prop to overwriteiosDiv
. - Use the
classIosSlot
prop to overwriteiosSlot
. - Use the
classIosTop
prop to overwriteiosTop
. - Use the
classIosLeftTop
prop to overwriteiosLeftTop
. - Use the
classIosLeftBot
prop to overwriteiosLeftBot
. - Use the
classIosRight
prop to overwriteiosRight
.
Name | Type | Default |
---|---|---|
iosTop | string | |
iosLeftTop | string | |
iosLeftBot | string | |
iosRight | string |
Laptop #
- Use the
classAndroidDiv
prop to overwriteandroidDiv
. - Use the
classAndroidDiv
prop to overwriteandroidDiv
. - Use the
classAndroidDiv
prop to overwriteandroidDiv
. - Use the
classAndroidDiv
prop to overwriteandroidDiv
. - Use the
classAndroidDiv
prop to overwriteandroidDiv
. - Use the
classAndroidDiv
prop to overwriteandroidDiv
.
Smartwatch #
- Use the
classAndroidDiv
prop to overwriteandroidDiv
. - Use the
classAndroidDiv
prop to overwriteandroidDiv
. - Use the
classAndroidDiv
prop to overwriteandroidDiv
. - Use the
classAndroidDiv
prop to overwriteandroidDiv
. - Use the
classAndroidDiv
prop to overwriteandroidDiv
. - Use the
classAndroidDiv
prop to overwriteandroidDiv
.
Name | Type | Default |
---|---|---|
smartRightTop | string | |
smartRightBot | string |
Tablet #
- Use the
classAndroidDiv
prop to overwriteandroidDiv
. - Use the
classAndroidDiv
prop to overwriteandroidDiv
. - Use the
classAndroidDiv
prop to overwriteandroidDiv
. - Use the
classAndroidDiv
prop to overwriteandroidDiv
. - Use the
classAndroidDiv
prop to overwriteandroidDiv
. - Use the
classAndroidDiv
prop to overwriteandroidDiv
.
Name | Type | Default |
---|---|---|
tabletLeftTop | string | |
tabletLeftMid | string | |
tabletLeftBot | string | |
tabletRight | string |